"required" finally makes Nullable types good
A bit of background. .NET has value types and reference types. Value types such as INT can not ever be null. If you type int myInt = null
then you will get a compile time error .NET then introduced nullable value types allowing you to create something like int? myInt
, and the compiler will force you to check if it is null before you can use it. Since any reference type could always be null there was no way to ever force them not to be null, and any usage could result in the dreaded "Object reference not set to an instance of an object" exception.
.NET core version 3 added support for C#8's Nullable reference types. To enable these project wide you would add the following to your csproj file
<PropertyGroup>
<Nullable>enable</Nullable>
<LangVersion>8</LangVersion>
</PropertyGroup>
This would force the compiler to also consider all reference types as non nullable unless specified with a "?" but this often meant either some dirty workarounds (public string Name { get; set; } = null!;
) or lots of boilerplate. e.g. to make the following POCO (plain old class object) null safe
class MyPoco
{
public string Name { get; set; }
public Address Address { get; set; }
}
We would need add more boilerplate code and always force it to be populated from a constructor
class MyPoco
{
public MyPoco(string name, Address address)
{
Name = name;
Address = address;
}
public string Name { get; set; }
public Address Address { get; set; }
}
Finally .NET 7 with C# Language version 11 introduced the required attribute!
We can now simply write:
class MyPoco
{
public required string Name { get; set; }
public required Address Address { get; set; }
}
And the compiler will force us to populate all the required fields
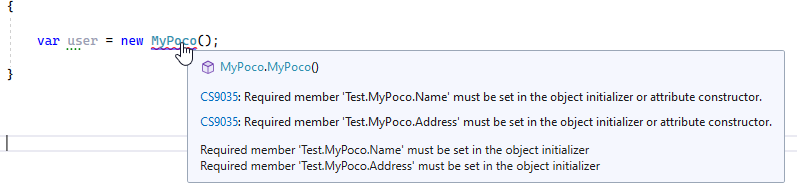